Recursive Objects
Madeup now supports recursion:
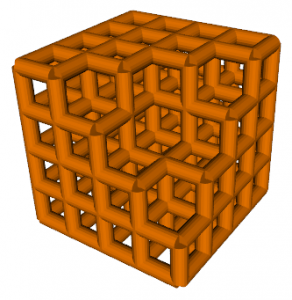
A recursively constructed 3-D lattice. One octant is missing at each level because I make only 7 recursive calls.
Including the function definition in the environment of a closure tripped me up considerably, but I think I got it figured out. Or maybe I didn’t.
The lattice was generated with this code:
radius = 0.1
nsides = 20
-- Draw a tube from point 1 to point 2.
line x1 x2 y1 y2 z1 z2 =
moveto x1 y1 z1
moveto x2 y2 z2
tube
end
-- Draw a box made of boxes.
box level x1 x2 y1 y2 z1 z2 =
if level > 0
-- Each subbox, or octant, will have one of its corners
-- touch the middle of this box.
midX = (x1 + x2) * 0.5
midY = (y1 + y2) * 0.5
midZ = (z1 + z2) * 0.5
box (level - 1) x1 midX y1 midY z1 midZ
box (level - 1) midX x2 y1 midY z1 midZ
box (level - 1) x1 midX midY y2 z1 midZ
box (level - 1) midX x2 midY y2 z1 midZ
box (level - 1) x1 midX y1 midY midZ z2
box (level - 1) midX x2 y1 midY midZ z2
box (level - 1) x1 midX midY y2 midZ z2
-- Let's omit the eighth call, for fun.
-- box (level - 1) midX x2 midY y2 midZ z2
else
-- X spanners
line x1 x2 y1 y1 z1 z1
line x1 x2 y2 y2 z1 z1
line x1 x2 y1 y1 z2 z2
line x1 x2 y2 y2 z2 z2
-- Y spanners
line x1 x1 y1 y2 z1 z1
line x2 x2 y1 y2 z1 z1
line x1 x1 y1 y2 z2 z2
line x2 x2 y1 y2 z2 z2
-- Z spanners
line x1 x1 y1 y1 z1 z2
line x2 x2 y1 y1 z1 z2
line x1 x1 y2 y2 z1 z2
line x2 x2 y2 y2 z1 z2
end
end
box 2 -1 1 -1 1 -1 1
Cleaning up the corners is a job for another day.