The Tiangle, Part I
This post is part of a series of notes and exercises for a summer camp on making musical instruments with Arduino and Pure Data.
Our First Instrument
It’s time to make our first instrument: the tiangle (sic). The tiangle will use a piece of hardware called a potentiometer, which is a knob that controls how many electrons pass through it. You’ll read the angle that it’s turned to set the frequency of the sound wave you generate with osc~
. And the notes will all be slurred or tied together. Thus, tiangle.
In this first exercise, we’ll build the Arduino half of the tiangle. It will make numbers, but not sound. In the next exercise, we’ll use Pure Data to read the numbers and produce the sound waves.
Hardware
The hardware of a tiangle looks something like this:
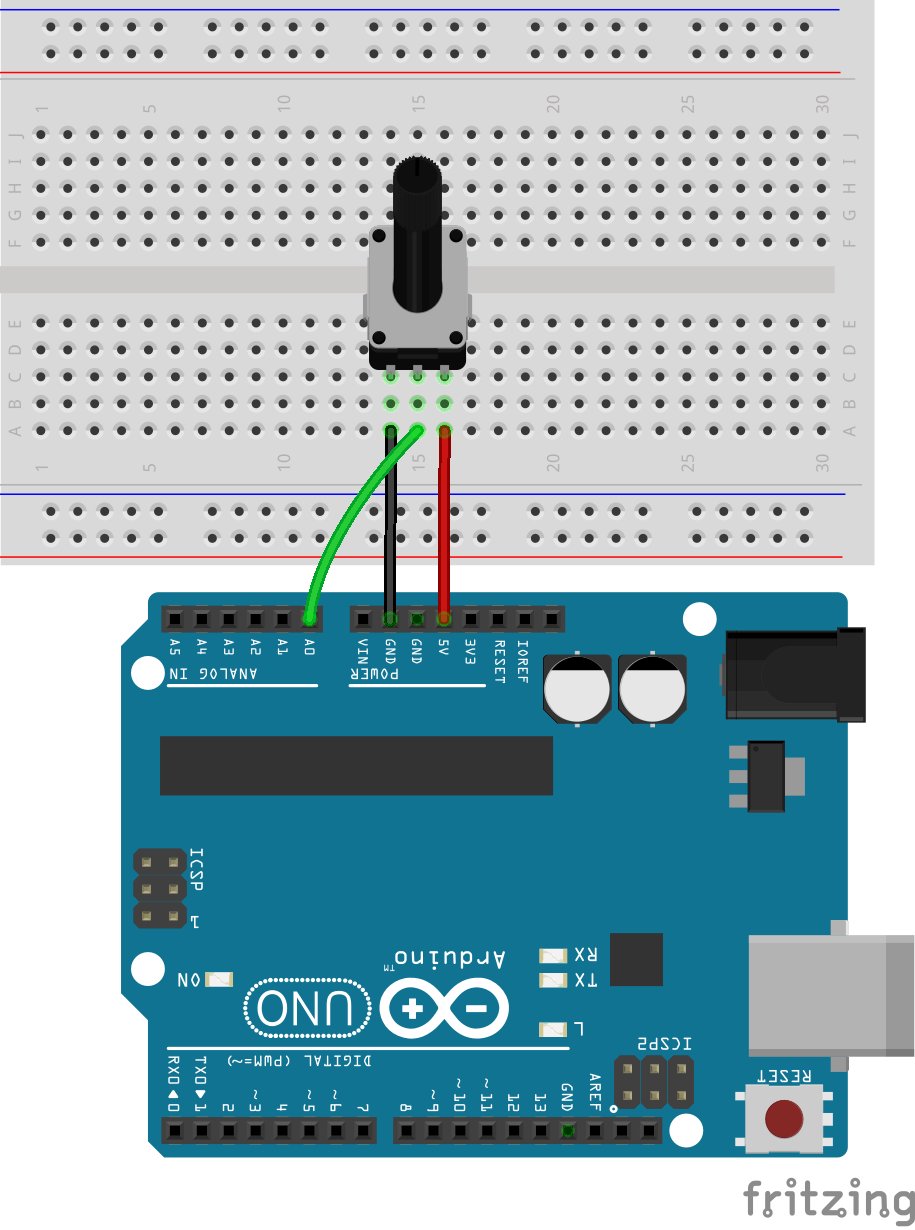
Electrons enter the circuit from ground and head to the left pin of the potentiometer. Some return back through to the 5-volt pin. Others sneak out the middle pin and head to A0, which stands for analog pin 0. Rotating the dial of the potentiometer one way lets more electrons through to A0. Rotating the other way lets fewer through.
Go ahead and assemble this circuit.
Firmware
Let’s now program the hardware to listen to pin A0 and report back to our computers the potentiometer’s angle. Open the Arduino development environment by hitting Command-Space
and typing Arduino in the box that appears.
Arduino programs are called sketches, and they are written in a language called C++. The Arduino development environment fires up a mostly blank sketch with two things in it:
void setup() {
// Code in here will get run exactly once when
// the Arduino is first powered on.
}
void loop() {
// Code in here will get run again and again.
}
Something that we want to do again and again is read from pin A0. We do that by invoking some code named analogRead
:
void loop() {
int reading = analogRead(A0);
}
With int reading
, we ask the Arduino to set aside enough space to store an integer. We refer to that space through the name reading
.
To send the reading from the Arduino to the computer, we push it across the USB cable using the Serial.println
function:
void loop() {
int reading = analogRead(A0);
Serial.println(reading);
}
Communicating over the serial port only works if both sender and receiver know how fast the data is being sent. We configure the speed on the Arduino side in setup
. Let’s send 9600 bits across the cable per second:
void setup() {
Serial.begin(9600);
}
Executing
Now it’s time to install our firmware onto our Arduino hardware. In the Arduino development environment, click the Verify button to compile your C++ code into the low-level machine code that the Arduino processor knows.
If it works, connect your Arduino to the computer using the USB cable. Select your Arduino under Tools / Port. It may be listed as SLAB_USBtoUART or usbmodem. Ask if you can’t find it. Hit Upload to send the machine code across the USB cable into the Arduino. If it doesn’t work, check your code closely. Make sure you’ve got all the correct punctuation and spelling.
Try turning the potentiometer. What happens? Probably nothing. That’s because we don’t have the Serial Monitor open. Click Tools / Serial Monitor. Set the speed to 9600 bits per second. You should start seeing readings! If not, seek help.
Challenges
After you get your sketch working, answer the following questions on a piece of scratch paper.
- What’s the range of readings that you can get from the potentiometer?
- Read about the
delay
function in the Arduino documentation. What number must you give it delay for a half second? Use it to slow down the stream of readings with a delay. - You can alter the value of
reading
with arithmetic like this, which happens to double it:Add some arithmetic to your program so that the Arduino emits numbers in the range [0, 255]. What was your arithmetic expression?int reading = analogRead(A0); reading = reading * 2; Serial.println(reading);